This blog provides you with the top 25 most frequently asked C++ interview questions and answers as of right now, along with novice level explanations.
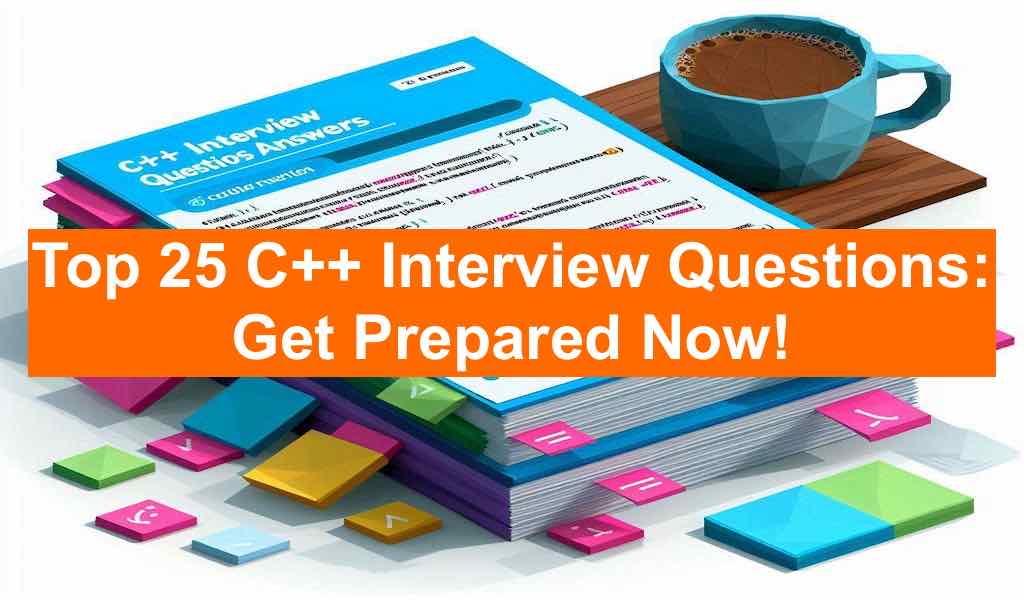
Table of Content
- 1. What is the difference between C and C++?
- 2. Explain the concept of object-oriented programming.
- 3. What are the access specifiers in C++ and what do they do?
- 4. What is a constructor? What are the different types of constructors?
- 5. What is the difference between stack and heap memory allocation?
- 6. Explain the concept of function overloading.
- 7. What is a virtual function?
- 8. How do 'reference' and 'pointer' vary from one another?
- 9. What is the 'const' keyword used for?
- 10. Explain the concept of function templates.
- 11. What is the Standard Template Library (STL)?
- 12. What distinguishes 'new' from'malloc()'
- 13. What is a 'destructor' and when is it called?
- 14. Explain the concept of 'operator overloading'.
- 15. What is a 'friend' function?
- 16. What is the difference between structs and classes in C++?
- 17. What is a pure virtual function?
- 18. What is an abstract class?
- 19. Explain the concept of multiple inheritance.
- 20. What is the difference between 'delete' and 'delete[]'?
- 21. What is a namespace?
- 22. What is the purpose of the 'mutable' keyword?
- 23. Explain the concept of exception handling in C++.
- 24. What is a smart pointer?
- 25. What distinguishes deep copy from shallow copy?
- Learn more about related and other topics
1. What is the difference between C and C++?
Answer: C++ is a powerful, general-purpose programming language that extends the C language. It adds features like classes, objects, and many other enhancements that make it suitable for both low-level system programming and high-level application development.
C++ is an extension of C with additional features like:
- Object-oriented programming (classes, objects, inheritance, polymorphism, encapsulation)
- Function overloading
- Exception handling
- Templates
- Standard Template Library (STL)
- References
- Improved type checking and memory management
2. Explain the concept of object-oriented programming.
Answer: Object-oriented programming (OOP) is a programming paradigm based on the concept of “objects” that contain data and code. The main principles of OOP are:
- Encapsulation: Combining within a single unit (class) data and methods that work with that data
- Inheritance: The ability for a class to take on attributes and functions from another class
- Polymorphism: The capacity of objects belonging to distinct classes to react differently to a same method call
- Abstraction: Keeping intricate implementation details hidden and displaying an object’s essential qualities only
3. What are the access specifiers in C++ and what do they do?
Answer: C++ has three access specifiers:
- public: Members are accessible from outside the class
- private: Members are only accessible within the class
- protected: Members are accessible within the class and by derived classes
4. What is a constructor? What are the different types of constructors?
Answer: A constructor is a special member function that is called when an object is created. It initializes the object’s data members. Types of constructors include:
- Default constructor: Takes no parameters
- Parameterized constructor: Takes parameters to initialize object members
- Copy constructor: Constructs an object by copying an already-existing one.
- Move constructor: Transfers ownership of resources from one object to another
5. What is the difference between stack and heap memory allocation?
Answer: Stack memory is used for local variables, while heap memory is used for dynamically allocated objects.
- Stack: Automatic memory allocation and deallocation. Faster but limited in size.
- Heap: Dynamic memory allocation and deallocation. Slower but larger in size.
6. Explain the concept of function overloading.
Answer: Function overloading allows multiple functions with the same name but different parameters (number, type, or order) to coexist in the same scope. -The arguments supplied to the compiler determine which function to invoke.
7. What is a virtual function?
Answer: A virtual function is a member function declared in a base class and redefined in a derived class. It’s used to achieve runtime polymorphism. When you refer to a derived class object using a pointer or reference to the base class, you can call a virtual function and execute the derived class’s version of the function.
8. How do ‘reference’ and ‘pointer’ vary from one another?
Answer:
- References must be initialized when declared; pointers can be initialized anytime
- References cannot be null; pointers can be null
- References cannot be reassigned; pointers can be reassigned
- References don’t have their own memory address; pointers have their own memory address
9. What is the ‘const’ keyword used for?
Answer: The ‘const’ keyword is used to declare constants or to specify that a variable’s value cannot be modified. It can be used with:
- Variables: To make them read-only
- Pointers: To make the pointer or the pointed value constant
- Member functions: To ensure they don’t modify the object’s state
10. Explain the concept of function templates.
Answer: Function templates allow you to write a function that can work with different data types without having to rewrite the function for each type. The compiler generates the appropriate function definitions at compile time based on the argument types provided.
11. What is the Standard Template Library (STL)?
Answer: The STL is a collection of powerful, reusable, and adaptable components in C++. It provides:
- Containers: such as vector, list, map
- Algorithms: such as sort, find, binary_search
- Iterators: to traverse container elements
- Function objects: items that are suitable for usage as parameters in functions
12. What distinguishes ‘new’ from’malloc()’
Answer:
- ‘malloc()’ is a function, whereas ‘new’ is an operator
- ‘new’ calls constructors, ‘malloc()’ doesn’t
- ‘malloc()’ returns void*, ‘new’ returns a typed pointer
- ‘new’ can be overloaded, ‘malloc()’ cannot
13. What is a ‘destructor’ and when is it called?
Answer: A destructor is a unique member function that is invoked in response to an object being destroyed. It’s used to clean up resources allocated by the object. Destructors are called:
- When an object goes out of scope
- When a dynamically allocated object is deleted
- At the end of the program for global and static objects
14. Explain the concept of ‘operator overloading’.
Answer: Operator overloading allows you to define how operators work with objects of user-defined classes. This is done by defining special member functions with the name operator followed by the symbol of the operator being overloaded.
15. What is a ‘friend’ function?
Answer: A friend function is a function that has access to the private and protected members of a class even though it is not a member of that class. It is declared inside the class with the friend keyword.
16. What is the difference between structs and classes in C++?
Answer: The main difference is the default access level:
- In structs, members are public by default
- In classes, members are private by default
Otherwise, they are functionally equivalent in C++.
17. What is a pure virtual function?
Answer: A pure virtual function is a virtual function that has no implementation in the base class and must be implemented by any concrete derived class. It’s declared using the syntax: virtual return_type function_name() = 0;
18. What is an abstract class?
Answer: An abstract class is a class that contains at least one pure virtual function. It cannot be instantiated and is used as a base class for other classes. Interfaces for derived classes are defined using abstract classes.
19. Explain the concept of multiple inheritance.
Answer: Multiple inheritance is when a class inherits from more than one base class. This can lead to the “diamond problem” when two base classes inherit from a common base class, which is resolved using virtual inheritance.
20. What is the difference between ‘delete’ and ‘delete[]’?
Answer:
- To deallocate memory for a single object, use the ‘delete’ command.
- ‘delete[]’ is used to deallocate memory for an array of objects
21. What is a namespace?
Answer: A namespace is a declarative region that provides a scope to the identifiers (names of types, functions, variables, etc.) inside it. Namespaces are used to avoid name clashes and arrange code logically into groups.
22. What is the purpose of the ‘mutable’ keyword?
Answer: The ‘mutable’ keyword allows a member of an object to be modified even if the object is declared as const. It’s typically used for caching or lazy evaluation scenarios.
23. Explain the concept of exception handling in C++.
Answer: Exception handling in C++ is done using try, catch, and throw statements:
- try: Contains the code that may throw an exception
- catch: Handles the exception if it occurs
- throw: Used to throw an exception when a problem occurs
24. What is a smart pointer?
Answer: Smart pointers are objects that function similarly to pointers but have extra features like memory management that happen automatically. C++ has three types of smart pointers:
- unique_ptr: For exclusive ownership
- shared_ptr: For shared ownership
- weak_ptr: A weak reference to an object managed by shared_ptr
25. What distinguishes deep copy from shallow copy?
Answer:
- Shallow copy: Copies the object’s memory address, resulting in two pointers to the same data
- Deep copy: Creates a new object and copies the old object’s data into it, resulting in two independent objects
Learn more about related and other topics
- Oracle Interview Questions: Must Know Before Your Big Day
- What Are the Most Common SQL Tricks Everyone Should Know?
- Are You Good Enough in PLSQL? Test your knowledge right now
- Python Interview Questions: A Quick Refresher for Intermediate
- Python Interview Questions: A Quick Refresher for Expert
- How to Answer Most Common Interview Questions: Part 4(2024)
- Learn C++ from Microsoft