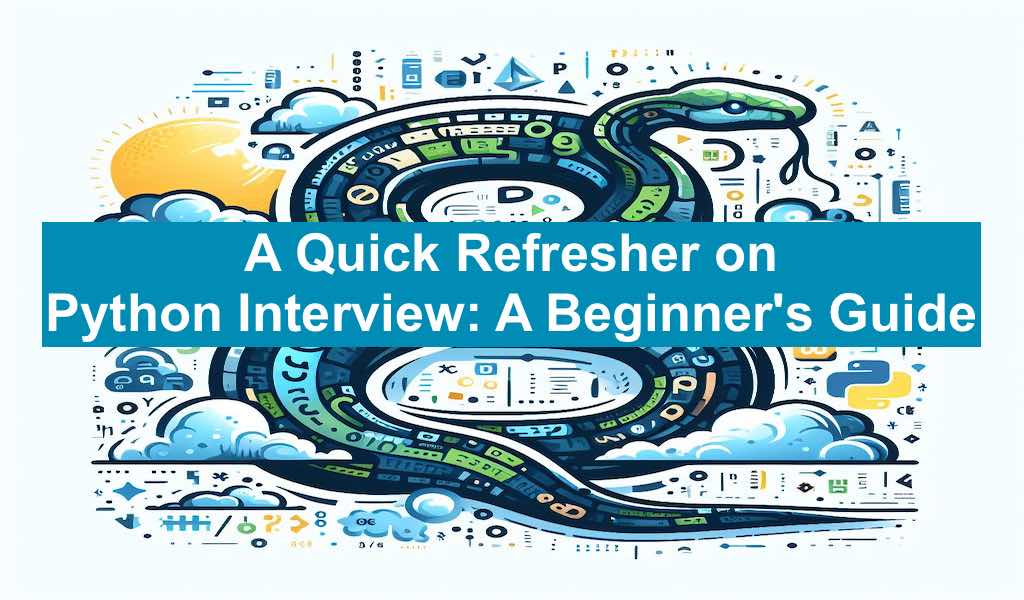
Explore the best Python interview questions and answers to help you land the position of your dreams in machine learning, data science, or Python coding.
Table of Contents
- What is Python?
- 1. List some applications of Python
- 2. Benefits of using Python
- 3. Is Python an interpreted or compiled language?
- 4. What does Python's "#" symbol mean?
- 5. How do mutable and immutable data types vary from one another?
- 6. What is the meaning of argument passed "by value" or "by reference" in Python?
- 7. How to distinguished between a Set and Dictionary?
- 8. What is List Comprehension? Give an Example.
- 9. What do you understand by lambda function?
- 10. What is "pass" does in Python?
- 11. Is there any difference between operator "/" and "//" in Python?
- 12. How is exceptional handling done in Python?
- 13. What swapcase() function does in Python?
- 14. What is the basic difference between "for loop" and "while loop" in Python?
- 15. Is it possible in Python to pass a function as an argument?
- 16. What does *args and *kwargs means in Python?
- 17. Is indentation required in Python?
- 18. What you understand with scope in Python?
- 19. What is "docstring" in Python and How it can be retrieve?
- 20. What do you understand with dynamically typed language?
- 21. What are the loop control statements in Python?
- 22. What do you understand by built-in data types in Python?
- 23. What floor() function is used for?
- Learn more about Python
What is Python?
One of the most extensively used and well-liked programming languages is Python, which Guido van Rossum created and was made available on February 20, 1991. Python is an open-source, free language with an extremely straightforward syntax that makes it simple for developers to learn. It is most frequently used for general-purpose programming and supports object-oriented programming. Python finds use in a multitude of fields, including Artificial Intelligence, Data Science, Machine Learning, Deep Learning, Scientific Computing, Networking, Game Development, Web Development, and Web Scraping, among others.
Python’s popularity is growing rapidly because of its simplicity and capacity to accomplish multiple tasks with a little number of lines of code. Owing to its capacity to facilitate robust computations through the use of robust libraries, Python finds application in diverse fields and has therefore led to an enormous need for Python developers worldwide. Businesses are eager to provide these developers with fantastic packages and perks.
1. List some applications of Python
- Machine learning and Artificial Intelligence
- System Scripting
- Web Development
- Game Development
- Software Development
- Complex Mathematics
- Web Scrapping
- Data Processing, Cleaning and Analysis
- Data Visualizations
2. Benefits of using Python
- Object-Oriented Language
- High-Level Language
- Dynamically Typed language
- Extensive support Libraries
- Presence of third-party modules
- Open source and community development
- Portable and Interactive
- Portable across Operating systems
3. Is Python an interpreted or compiled language?
Python is actually a partially interpreted and partially compiled language. When we run our code, the compilation process begins. This generates byte code internally, which the Python virtual machine (p.v.m) then converts to the appropriate format depending on the underlying platform (computer + operating system).
4. What does Python’s “#” symbol mean?
The symbol ‘#’ is used to add a comment. Anything comes after ‘#’ in that line is considered as comment.
5. How do mutable and immutable data types vary from one another?
Data types that can be modified after it has created are considered as mutable data types. Eg – List, Set, Dictionary, etc.
Data types that cannot be modified after it has created are considered as immutable data types. Eg – String, Tuple, etc.
6. What is the meaning of argument passed “by value” or “by reference” in Python?
In Python, everything is an object, and references to those objects are stored in variables. You are unable to alter the value of the references since they are set by the functions. On the other hand, if an object is mutable, you can alter it.
7. How to distinguished between a Set and Dictionary?
The set is an unordered, changeable, iterable collection of data types without duplicates.
In Python, a dictionary is an ordered set of data values that is similar to a map in terms of storage.
8. What is List Comprehension? Give an Example.
Python’s list comprehension module offers a straightforward and condensed syntax for turning any other iterable—such as a string or another list—into a list.
Syntax

For Example:
my_list = [i for i in range(1, 10)]
9. What do you understand by lambda function?
A lambda function is an anonymous function.
Syntax

A lambda function can have a single statement but can have any number of arguments. For Example:
a = lambda x, y : x*y print(a(10, 200))
10. What is “pass” does in Python?
Pass indicates that no action is taken, or to put it another way, it is a statement for placeholder where nothing needs to be stated and there should be a blank left.
11. Is there any difference between operator “/” and “//” in Python?
Operator “//” denotes floor division, it means we only gets integer part of the result. Whereas operator “/” denotes actual division with decimal points. For Example:
7//2 = 3 7/2 = 3.5
12. How is exceptional handling done in Python?
Try, except, and finally are the three primary keywords that are used to capture exceptions and adjust the recovering mechanism accordingly. The code block called “try” is the one that is checked for mistakes. The “except” block is only executed in the event of an error.
The “finally” block’s charm lies in its ability to run the code after attempting an error. Whether an error happened or not, this block is still run. These blocks are utilized to do the necessary cleanup tasks for variables and objects.
13. What swapcase() function does in Python?
This method takes a string and changes every uppercase character to lowercase and vice versa. It is used to change the string’s current case. By using this function, a new copy of the string with every character in the swap case is created. As an illustration see the below example:
string = "TechyBuddy" string.swapcase() ---> "tECHYbUDDY"
14. What is the basic difference between “for loop” and “while loop” in Python?
Iterating through the items of different collection types, such as List, Dictionary, Tuple, and Set, is typically done using the “for” loop. When creating a “for” loop, developers provide the criteria at the beginning and the end. On the other hand, the real looping functionality found in all other programming languages is the “while” loop. Python while loops are used by programmers when they only know conditions to the end the loop.
15. Is it possible in Python to pass a function as an argument?
It is possible to pass a function with several parameters, including variables, objects, and functions (of the same or other data types). As functions are object too it is possible to be passed as parameters to other functions. Functions that accept other functions as a parameter are known as higher-order functions.
16. What does *args and *kwargs means in Python?
We use the unique syntax *args and **kwargs in the function specification to pass a variable number of arguments to a Python function. It is used to pass a variable-length, keyword-free argument list. The variable becomes iterable once it is associate with the *, enabling you to perform any operation that you can perform on any iterables, including higher-order operations like map and filter, as well as iterating over it.
17. Is indentation required in Python?
Yes, indentation is necessary in Python. Python indentation can be used to tell a Python interpreter that a set of statements is part of a certain block of code. In many programming languages, indentations make the code easier to read for developers; nevertheless, in Python, it’s crucial to indent the code in a particular order.
18. What you understand with scope in Python?
The scope of a variable is the place where we may locate it and access it when needed.
- Local variable: Local variables are specific to a function and are initialized only inside of it. It is not accessible from outside the function.
- Global variables: These are variables that are declared and defined outside of functions and are not specific to any one function.
- Module-level scope: This describes the program’s current module’s global items that are accessible.
- Outermost scope: This describes any built-in names that the application is capable of calling. Among all the scope, this scope is searched last.
19. What is “docstring” in Python and How it can be retrieve?
We can associate documentation with Python modules, functions, classes, and methods is made easy with the help of Python documentation strings, or docstrings.
- Declaration of Docstrings: The declaration of the docstrings comes directly after the declaration of the class, method, or function. It is done by using “triple single quotes” or “triple double quotes.” There should be a docstring for every function.
- Accessing Docstrings: The help function or the object’s __doc__ method can be used to access the docstrings.
20. What do you understand with dynamically typed language?
Typed languages are those in which the data type is defined and is recognized by the compiler/machine either at compilation or during runtime. Two categories can be used to group typed languages:
- Statically typed languages: Languages with statically typed, data type of each variable should be known at compile time which require programmer should provide the data type of each variable at the time of variable declaration
- Dynamically typed languages: These are languages in which variables have no need of a predefined data type because the machine interprets them as needed. In these languages, interpreters determine a variable’s data type based on its value at runtime.
21. What are the loop control statements in Python?
The purpose of the break statement is to end the current loop that contains it. The statement that is present after the loop statement, if any, control will then resume from that statement.
The continue statement is also a loop control statement. The continue statement acts in the opposite way as the break statement, forcing the loop to go on to the next iteration rather than ending it.
Pass is equivalent to doing nothing (no operation); it is a placeholder in the compound statement where nothing needs to be done and one statement is required for correcting the syntax.
22. What do you understand by built-in data types in Python?
The standard or built-in data types in Python are as follows:
- Numeric: In Python, data with a numeric value is represented by the numeric data type. An integer, floating point, Boolean, or even complex number can all be considered numerical values.
- Sequence Type: Python defines a sequence data type as an ordered collection of either distinct or similar data types. Python has multiple sorts of sequences:
- String
- List
- Tuple
- Python range
- Mapping Types: A mapping object in Python allows hashable data to be mapped to arbitrary objects. At the moment, the dictionary is the sole common mapping type and is a mutable type.
- Python Dictionary
- Set Types: An unordered, changeable, iterable collection of data types with no duplicate items is referred as a set in Python. Even though a set may contain a variety of elements, the order in which they appear is not specified.
23. What floor() function is used for?
The floor() function is a function from the math module and can be utilized to find the floor of a number.
- floor(n) function returns the floor of ‘n’ i.e., the largest integer not greater than ‘n’.
- Also there is another related function is ceil(n) in math module that returns a ceiling value of ‘n’ i.e., the smallest integer greater than or equal to ‘n’
Learn more about Python
- Python Interview Questions: A Quick Refresher for Intermediate
- Python Interview Questions: A Quick Refresher for Expert
- Test Your Knowledge on Python Lists
- List Comprehensions by Python.org
- Python Core Concepts in 10 Mins
- Python List Comprehension: Get Your Code Simplified Today