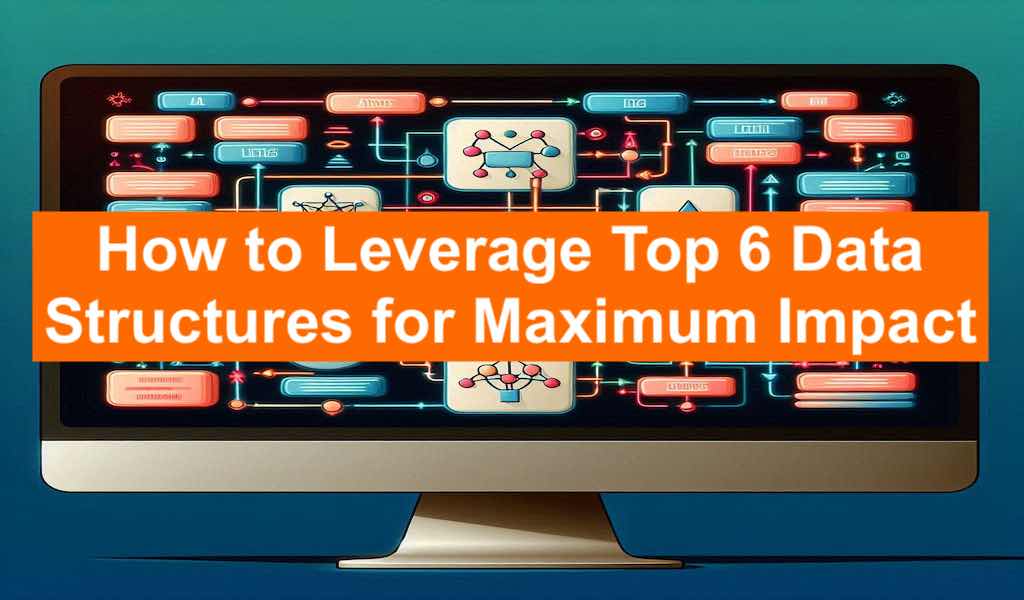
Dive into the world of Data Structures with our comprehensive guide for programmers of all levels. Explore arrays, linked lists, trees, graphs, and more through beginner-friendly explanations, practical examples, and real-world applications. Unlock the secrets to efficient coding, optimize your algorithms, and level up your programming skills. From basic concepts to advanced implementations, this guide covers it all, complete with pros, cons, and FAQs for each data structure. Whether you’re a coding newbie or a seasoned developer, discover how mastering data structures can transform your approach to problem-solving and elevate your software engineering prowess.
Table of Content
- Introduction to Data Structures
- Types of Data Structures
- The Role of Data Structures in Algorithms
- Common Data Structures and Their Uses
- Choosing the Right Data Structure
- Practical Implementation and Best Practices
- Conclusion
- FAQs
- Q1. What is the most commonly used data structure?
- Q2. How do I choose the right data structure for my project?
- Q3. Can I use multiple data structures in a single program?
- Q4. What resources are available for learning more about data structures?
- Q5. How do data structures impact the performance of my code?
- Q6. When should I use an array?
- Q7. Can I resize an array?
- Q8. What's the difference between singly and doubly linked lists?
- Q9. When should I use a linked list instead of an array?
- Q10. What's the difference between a tree and a graph?
- Q11. How are graphs used in social networks?
- Q12. What happens if two keys hash to the same index?
- Q13. When should I use a hash table instead of an array?
- Q14. What's the difference between a binary tree and a binary search tree?
- Q15. How do trees help in file systems?
- Q16. Can I implement a stack using an array?
- Q17 What's a real-world example of a queue?
- Learn more about related or other topics
Introduction to Data Structures
Hey there, aspiring code wizards! Today, we’re diving into the fascinating world of data structures. Don’t worry if you’re new to this – we’ll break it down step by step and make it as fun as possible. So, grab your favorite beverage, get comfy, and let’s explore the building blocks that make our programs efficient and powerful!
What Are Data Structures?
Imagine you’re organizing a huge library. You wouldn’t just throw all the books in a pile, right? You’d want to sort them by genre, author, or maybe even color (if you’re feeling fancy). That’s exactly what data structures do for our programs – they help us organize and manage data efficiently.
Definition
Data structures are specialized formats for organizing, processing, retrieving, and storing data in a computer program.
Purpose
They make it easier to access and work with data, ultimately making our programs faster and more efficient.
Real-world analogy
Think of data structures as different types of containers. Arrays are like egg cartons (fixed size, easy access), linked lists are like chains (flexible size, but you need to follow the links), and trees are like family trees (hierarchical organization).
Types of Data Structures
There are several ways to categorize data structures:
Primitive vs. Non-primitive
- Primitive: Built-in types like integers, floats, and characters.
- Non-primitive: Custom structures like arrays, linked lists, and trees.
Linear vs. Non-linear
- Linear: Data arranged in a sequence (arrays, linked lists).
- Non-linear: Data with multiple connections (trees, graphs).
Static vs. Dynamic
- Static: Fixed size (arrays).
- Dynamic: Can grow or shrink as needed (linked lists, dynamic arrays).
The Role of Data Structures in Algorithms
Data structures and algorithms go together like peanut butter and jelly. The right data structure can make an algorithm super efficient, while the wrong one can slow it down to a crawl.
Example: Imagine you’re searching for a word in a dictionary. If the words are in random order (unorganized data structure), you’d have to check every single word. But with an alphabetically sorted dictionary (organized data structure), you can use a much faster binary search algorithm.
Common Data Structures and Their Uses
Arrays
Definition: An array is a collection of elements stored at contiguous memory locations.
Index: 0 1 2 3
Array: [apple, banana, cherry, date]
Example:
fruits = ["apple", "banana", "cherry", "date"]
print(fruits[1]) # Output: banana
Benefits:
- Fast access to elements using indices
- Simple and easy to use
- Efficient for iterating through all elements
Distinct features:
- Fixed size (in most languages)
- Elements are stored in contiguous memory
How it differs from others:
- Unlike linked lists, arrays provide constant-time access to elements
- Less flexible than dynamic data structures
Pros:
- Quick element access
- Memory efficient for a known, fixed number of elements
Cons:
- Fixed size in many languages (can’t easily add or remove elements)
- Inefficient for inserting or deleting elements in the middle
Linked Lists
Definition: A linked list is a linear data structure where elements are stored in nodes, and each node has its own data and points to the next node in the sequence.
[Head] -> [apple | Next] -> [banana | Next] -> [cherry | Next] -> [Null]
Example:
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
return
current = self.head
while current.next:
current = current.next
current.next = new_node
# Usage
ll = LinkedList()
ll.append("apple")
ll.append("banana")
ll.append("cherry")
print(ll.head.next.data) #print banana
print(ll.head.data) #print apple
Benefits:
- Dynamic size (can grow or shrink easily)
- Efficient insertion and deletion of elements
Distinct features:
- Nodes contain data and a reference to the next node
- Can be singly linked (one-way) or doubly linked (two-way)
How it differs from others:
- More flexible than arrays for adding/removing elements
- Requires more memory than arrays due to storing references
Pros:
- Easy insertion and deletion of elements
- Dynamic size
Cons:
- Slower access to individual elements (need to traverse the list)
- More memory overhead compared to arrays
Stacks and Queues
Stacks and queues are special types of linear data structures that follow specific rules for adding and removing elements.
Stack:
Definition: A stack follows the Last-In-First-Out (LIFO) principle.
Top
|
[apple] <- Push(apple)
[banana] <- Push(banana)
[cherry] <- Push(cherry)
Example:
stack = []
stack.append("apple") # Push
stack.append("banana")
stack.append("cherry")
print(stack.pop()) # Pop - Output: cherry
Queue:
Definition: A queue follows the First-In-First-Out (FIFO) principle.
Front Rear
| |
[apple] -> [banana] -> [cherry]
Example:
from collections import deque
queue = deque()
queue.append("apple") # Enqueue
queue.append("banana")
queue.append("cherry")
print(queue.popleft()) # Dequeue - Output: apple
Benefits:
- Stacks: Useful for tracking function calls, undo operations, and parsing expressions
- Queues: Great for managing tasks, breadth-first search algorithms, and scheduling
Distinct features:
- Stacks: Push (add) and pop (remove) operations occur at the same end
- Queues: Enqueue (add) at one end, dequeue (remove) from the other end
How they differ:
- Stacks reverse the order of elements, while queues maintain the original order
Pros:
- Simple and efficient for their specific use cases
- Easy to implement and understand
Cons:
- Limited functionality compared to more general-purpose data structures
- Not suitable for random access or complex operations
Trees
Definition: A tree is a hierarchical data structure consisting of nodes connected by edges.
[5]
/ \
[3] [7]
/ \ \
[1] [] [9]
Example (Binary Search Tree):
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, value):
if not self.root:
self.root = Node(value)
else:
self._insert_recursive(self.root, value)
def _insert_recursive(self, node, value):
if value < node.value:
if node.left is None:
node.left = Node(value)
else:
self._insert_recursive(node.left, value)
else:
if node.right is None:
node.right = Node(value)
else:
self._insert_recursive(node.right, value)
# Usage
bst = BinarySearchTree()
bst.insert(5)
bst.insert(3)
bst.insert(7)
bst.insert(1)
bst.insert(9)
print(bst.root.value) #print 5
print(bst.root.left.value) # print 3
Benefits:
- Efficient searching, insertion, and deletion operations
- Naturally represent hierarchical relationships
Distinct features:
- Nodes can have multiple children
- Various types: binary trees, binary search trees, AVL trees, etc.
How it differs from others:
- Non-linear structure, unlike arrays and linked lists
- Allows for efficient searching in logarithmic time (for balanced trees)
Pros:
- Fast search, insert, and delete operations (for balanced trees)
- Represent hierarchical data naturally
Cons:
- More complex to implement than linear data structures
- Balancing trees can be challenging
Graphs
Definition: A graph is a non-linear data structure consisting of vertices (nodes) and edges that connect these vertices.
Nodes: {A, B, C, D}
Edges: {(A, B), (A, C), (B, D), (C, D)}
A
/ \
B C
\ /
D
Example:
class Graph:
def __init__(self):
self.graph = {}
def add_edge(self, u, v):
if u not in self.graph:
self.graph[u] = []
self.graph[u].append(v)
# Usage
g = Graph()
g.add_edge(0, 1)
g.add_edge(0, 2)
g.add_edge(1, 2)
g.add_edge(2, 0)
g.add_edge(2, 3)
g.add_edge(3, 3)
print(g.graph) # prints {0: [1, 2], 1: [2], 2: [0, 3], 3: [3]}
Benefits:
- Model complex relationships and networks
- Solve real-world problems like finding shortest paths or detecting cycles
Distinct features:
- Can be directed or undirected
- May have weighted edges
How it differs from others:
- More flexible than trees (can have cycles)
- Can represent any kind of relationship between entities
Pros:
- Versatile for modeling various types of relationships
- Many powerful algorithms available (e.g., Dijkstra’s, A* search)
Cons:
- Can be complex to implement and manage
- Some graph algorithms can be computationally expensive
Hash Tables
Definition: A hash table is a data structure that implements an associative array abstract data type, a structure that can map keys to values.
{ key1: value1, key2: value2, key3: value3 }
Example:
# In Python, dictionaries are implemented as hash tables
phone_book = {}
phone_book["Alice"] = "123-456-7890"
phone_book["Bob"] = "987-654-3210"
print(phone_book["Alice"]) # Output: 123-456-7890
Benefits:
- Very fast lookup, insertion, and deletion operations
- Efficient for large datasets
Distinct features:
- Uses a hash function to compute an index for each key
- Handles collisions through various methods (e.g., chaining, open addressing)
How it differs from others:
- Provides average-case constant time complexity for basic operations
- Not ordered like arrays or linked lists
Pros:
- Extremely fast access for large datasets
- Flexible key types (not just integers)
Cons:
- Can have poor performance if the hash function is not well-designed
- May require more memory than other data structures
Choosing the Right Data Structure
Selecting the appropriate data structure is crucial for writing efficient programs. Here are some factors to consider:
Factors to Consider
- Time complexity: How fast are the operations you need?
- Space complexity: How much memory can you afford to use?
- Nature of the data: Is it static or dynamic? Does it need to be ordered?
- Required operations: Do you need fast insertions, deletions, or lookups?
Common Scenarios and Recommendations
- Need fast lookups by index? Use an array.
- Frequent insertions/deletions at the beginning or middle? Consider a linked list.
- Need to maintain a sorted collection? Try a binary search tree.
- Working with graph-like relationships? Use a graph structure.
- Need fast key-value lookups? Hash table is your friend.
Performance Trade-offs
Remember, there’s often a trade-off between time and space complexity. For example, hash tables offer fast lookups but may use more memory than a simple array.
Practical Implementation and Best Practices
Coding Data Structures from Scratch
- Start with simpler structures like linked lists and work your way up
- Always consider edge cases (empty structures, single elements, etc.)
- Use unit tests to verify your implementations
Utilizing Built-in Data Structures
- Most programming languages offer efficient built-in implementations
- For example, Python’s list is a dynamic array, and dict is a hash table
- Use these when possible for better performance and reliability
Best Practices
- Document your code, especially for custom implementations
- Keep your data structures modular and reusable
- Stay updated with new developments in data structure theory
Conclusion
Whew! We’ve covered a lot of ground, from the basics of arrays to the complexities of graphs and hash tables. Remember, mastering data structures is a journey, not a destination. The more you practice and experiment, the better you’ll become at choosing and implementing the right structures for your programs.
Don’t be afraid to dive deeper into each of these topics. There’s always more to learn, and every great programmer started exactly where you are now. Keep coding, keep learning, and most importantly, have fun with it! Remember, the best way to learn is by doing. Try implementing these data structures yourself, and don’t be afraid to experiment with different approaches. Happy coding!
FAQs
Q1. What is the most commonly used data structure?
Arrays and hash tables (dictionaries in some languages) are among the most commonly used data structures due to their versatility and efficiency for many tasks.
Q2. How do I choose the right data structure for my project?
Consider the operations you’ll perform most often, the nature of your data, and the time and space complexity requirements of your project. When in doubt, start with a simple structure and optimize as needed.
Q3. Can I use multiple data structures in a single program?
Absolutely! In fact, many complex programs use a combination of data structures to handle different aspects of data management efficiently.
Q4. What resources are available for learning more about data structures?
There are many great resources available, including online courses (like those on Coursera or edX), textbooks (such as “Introduction to Algorithms” by Cormen et al.), and coding practice websites like LeetCode or HackerRank.
Q5. How do data structures impact the performance of my code?
Data structures can significantly impact both the time and space efficiency of your code. Choosing the right data structure can make your program run faster and use less memory, especially when dealing with large amounts of data.
Q6. When should I use an array?
Use arrays when you know the number of elements in advance and need fast access to elements by their position.
Q7. Can I resize an array?
In most languages, arrays have a fixed size. However, some languages offer dynamic arrays (like ArrayList in Java or List in Python) that can be resized.
Q8. What’s the difference between singly and doubly linked lists?
Singly linked lists only have references to the next node, while doubly linked lists have references to both the next and previous nodes.
Q9. When should I use a linked list instead of an array?
Use linked lists when you need frequent insertions or deletions, especially at the beginning or middle of the list, and when you don’t need random access to elements.
Q10. What’s the difference between a tree and a graph?
A tree is a special type of graph with no cycles and a single root node.
Q11. How are graphs used in social networks?
In social networks, vertices represent users, and edges represent connections or friendships between users.
Q12. What happens if two keys hash to the same index?
This is called a collision. Hash tables use various methods to resolve collisions, such as chaining (using linked lists at each index) or open addressing (finding the next available slot).
Q13. When should I use a hash table instead of an array?
Use a hash table when you need fast lookups based on arbitrary keys, not just integer indices, or when dealing with a large, sparsely populated set of possible keys.
Q14. What’s the difference between a binary tree and a binary search tree?
In a binary search tree, the left child is always smaller than the parent, and the right child is always larger, which enables efficient searching.
Q15. How do trees help in file systems?
File systems use tree structures to organize directories and files, making it easy to navigate and manage hierarchical data.
Q16. Can I implement a stack using an array?
Yes, you can implement a stack using an array by adding and removing elements from one end.
Q17 What’s a real-world example of a queue?
A printer queue is a great example – print jobs are processed in the order they were received.
Learn more about related or other topics
- Data Warehouse: A Beginner’s Guide To The New World
- Snowflake: How to Leverage for Maximum Efficiency (2024)
- What’s new in Oracle 19c and how does its architecture work?
- Oracle Definer Rights Vs Invokers Right: How To Choose?
- Snowflake Time Travel: How to Make It Work for You?
- NoSQL Vs SQL Databases: An Ultimate Guide To Choose
- AWS Redshift Vs Snowflake: How To Choose?
- Oracle Live SQL: How To Use Oracle Without Downloading?
- Computer Algorithms by Khan Academy