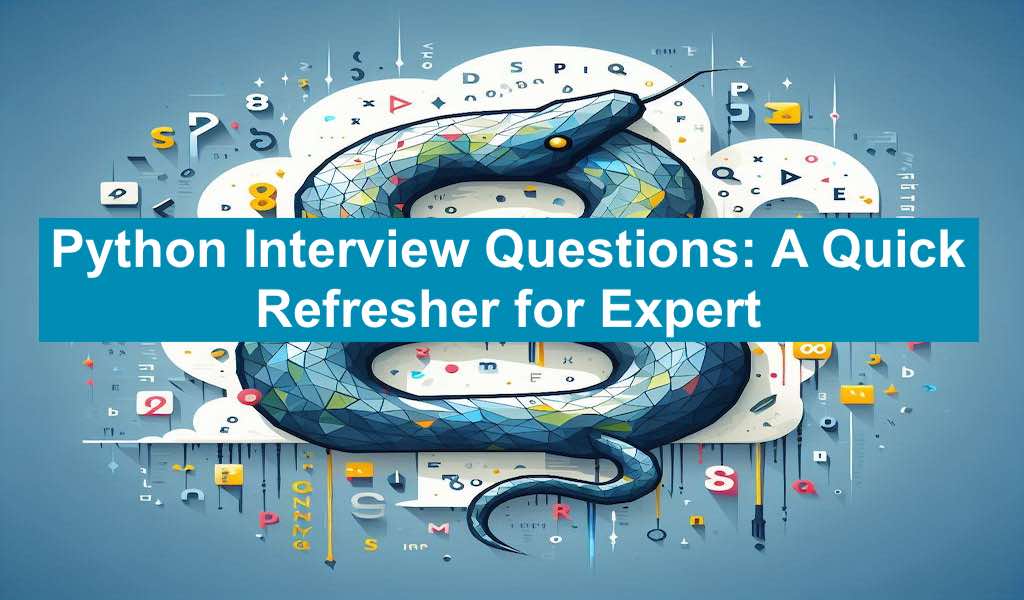
Python interviews assess your knowledge of Python, data structures, libraries, and problem-solving skills. Prepare well with our handpicked questions for Expert.
Table of Contents
- 1. What is PIP?
- 2. What is a zip function?
- 3. What are Pickling and Unpickling?
- 4. What is monkey patching in Python?
- 5. What is __init__() in Python?
- 6. Write a code to display the current time?
- 7. What are Access Specifiers in Python?
- 8. What are unit tests in Python?
- 9. What is Global Interpreter Lock (GIL)?
- 10. What are Function Annotations?
- 11. What are Exception Groups?
- 12. What is Switch Statement
- 13. What is virtualenvs?
- 14. What’s the difference between local and global namespaces?
- 15. How you can describe compile-time and run-time code checking in Python
- 16. Differentiate instance and class variables
- Learn more on Python
1. What is PIP?
The Python Installer Package, or PIP for short, offers a smooth interface for installing different Python modules. It’s a command-line utility that can look up packages online and install them without requiring user input.
# Unix/macOS
# Check if pip is installed and available, if not you may need to check if pip path is accessable
python3 -m pip --version
# installing a package
python3 -m pip install "SomeProject"
# Specific package version
python3 -m pip install "SomeProject==1.4"
# Any version greater than specified
python3 -m pip install "SomeProject~=1.4.2"
# install packages lists in a requirement file
python3 -m pip install -r requirements.txt
# Windows
# Check if pip is installed and available, if not you may need to check if pip path is accessable
py -m pip --version
# installing a package
py -m pip install "SomeProject"
# Specific package version
py -m pip install "SomeProject==1.4"
# Any version greater than specified
py -m pip install "SomeProject~=1.4.2"
# install packages lists in a requirement file
py -m pip install -r requirements.txt
2. What is a zip function?
A zip() function provides a zip object, which maps a comparable index of several iterables. It starts with an iterable, turns it into an iterator, and then aggregates the elements according to the passed iterables. An iterator of tuples is returned.

*iterables
: Represents one or more iterable objects (e.g., lists, tuples, strings).- Returns an iterator that pairs elements from corresponding positions in the input iterables.
fruits = ['Apple', 'Mango', 'Banana']
costs = [200, 100, 60]
zipped = zip(fruits, costs)
print(list(zipped))
# Output: [('Apple', 200), ('Mango', 100), ('Banana', 60)]
3. What are Pickling and Unpickling?
Pickling is the process by which the Pickle module takes any Python object, transforms it into a string representation, and uses the dump method to dump it into a file. Unpickling, on the other hand, is the act of recovering the original Python objects from the string representation that has been stored.
4. What is monkey patching in Python?
The phrase “monkey patch” in Python exclusively describes run-time, dynamic changes made to a class or module.
# tb.py class TechyBuddyClass: def function(self): print "Called Class function()" import tb def modified_function(self): print "Called modified_function()" tb.TechyBuddyClass.function = modified_function obj = TechyBuddyClass() obj.function() # Output Called modified_function()
5. What is __init__() in Python?
In terms of OOP, __init__ is a reserved method in Python classes that is comparable to constructors. The __init__ method is invoked automatically upon the creation of a new object. As soon as the new object is formed, memory is allocated to it using this method. Variable initialization can also be accomplished with this method.
6. Write a code to display the current time?
currenttime= time.localtime(time.time()) print (“Current time is”, currenttime)
7. What are Access Specifiers in Python?
Python determines the access control for a particular data member or member function of a class using the ‘_’ symbol. Three different kinds of Python access modifiers are available for a class:
- Public Access Modifier: When a class is made public, anyone can readily access its members from anywhere inside the program. A class’s member methods and data members are all by default public.
- Protected Access Modifier: Only classes that are derived from a class that has its members specified as protected can access them. A single underscore (‘_’) symbol is placed before each member of a class to indicate that all of its members are protected.
- Private Access Modifier: The most secure access modifier is the private access modifier. Members of a class that are designated as private can only be accessed within the class. A double underscore “__” sign is added before a data member of a class to indicate that the member is private.
8. What are unit tests in Python?
The smallest testable components of the product are tested at the first stage of software testing, known as unit testing. This is done to ensure that every software unit operates as intended. Python’s xUnit-style framework serves as the unit test framework. Unit testing is done using the White Box Testing approach.
9. What is Global Interpreter Lock (GIL)?
The Global Interpreter Lock (GIL) is a type of process lock that is used by Python whenever it deals with processes. Generally, Python uses only one thread to execute the set of written statements. The performance of the single-threaded process and the multi-threaded process will be the same in Python and this is because of GIL. Python lacks multithreading because of a global interpreter lock that limits the number of threads and makes each thread function as a single thread.
10. What are Function Annotations?
You can add metadata to function parameters and return values using the function annotation feature. In this manner, the return type of the value the function returns and the input type of the function parameters can both be specified.
Function annotations are actually random Python expressions that are linked to different sections of functions. These expressions have no life in the Python runtime environment; they are evaluated at build time. Python gives these annotations no significance.
11. What are Exception Groups?
Exception Groups is the most recent addition to Python 3.11. A new except* syntax can be used to handle the ExceptionGroup. Each except* clause can handle more than one exception, as indicated by the * sign.
A collection or group of various exception types is called an ExceptionGroup. The sequence in which the exceptions are saved in the Exception Group doesn’t matter when calling them; instead, we can group together various exceptions that we can later collect one at a time as needed.
try : raise ExceptionGroup( 'Example ExceptionGroup' , ( TypeError( 'Example TypeError' ), ValueError( 'Example ValueError' ), KeyError( 'Example KeyError' ), AttributeError( 'Example AttributeError' ) )) except * TypeError: ... except * ValueError as e: ... except * (KeyError, AttributeError) as e: ... |
12. What is Switch Statement
Python has included a switch case feature known as “structural pattern matching” starting with version 3.10. This feature can be used with the match and case keywords. Keep in mind that in Python, you set a default case for the switch statement using the underscore symbol.
Note: Before Python 3.10, match statements doesn’t support.
match term: case pattern - 1 : action - 1 case pattern - 2 : action - 2 case pattern - 3 : action - 3 case _: action - default |
13. What is virtualenvs?
- A virtualenv is an isolated environment for developing, running, and debugging Python code.
- It allows you to isolate a Python interpreter along with a specific set of libraries and settings.
- With
pip
, you can develop, deploy, and run multiple applications on a single host, each with its own Python interpreter version and separate libraries
14. What’s the difference between local and global namespaces?
- Local namespace: Exists within a function or method. Variables defined here are temporary and only accessible within that scope.
- Global namespace: Exists at the module level. Variables defined here are accessible throughout the entire module.
- Understanding scoping rules is crucial for avoiding naming conflicts and managing variable lifetimes
15. How you can describe compile-time and run-time code checking in Python
- Compile-time: Python is an interpreted language, so there’s no separate compilation step. Syntax errors are caught during parsing.
- Run-time: Errors like division by zero, attribute errors, etc., are detected during program execution
16. Differentiate instance and class variables
- Instance variables: Belong to an instance of a class. Each object has its own copy.
- Class variables: Shared among all instances of a class. Defined at the class level.
- Class variables are useful for storing data common to all instances.
Learn more on Python
- Test Your Knowledge on Python Lists
- A Quick Refresher on Python Interview: A Beginner’s Guide
- Python Interview Questions: A Quick Refresher for Intermediate
- Python Core Concepts in 10 Mins
- Learn Python on Official Website
- Python List Comprehension: Get Your Code Simplified Today