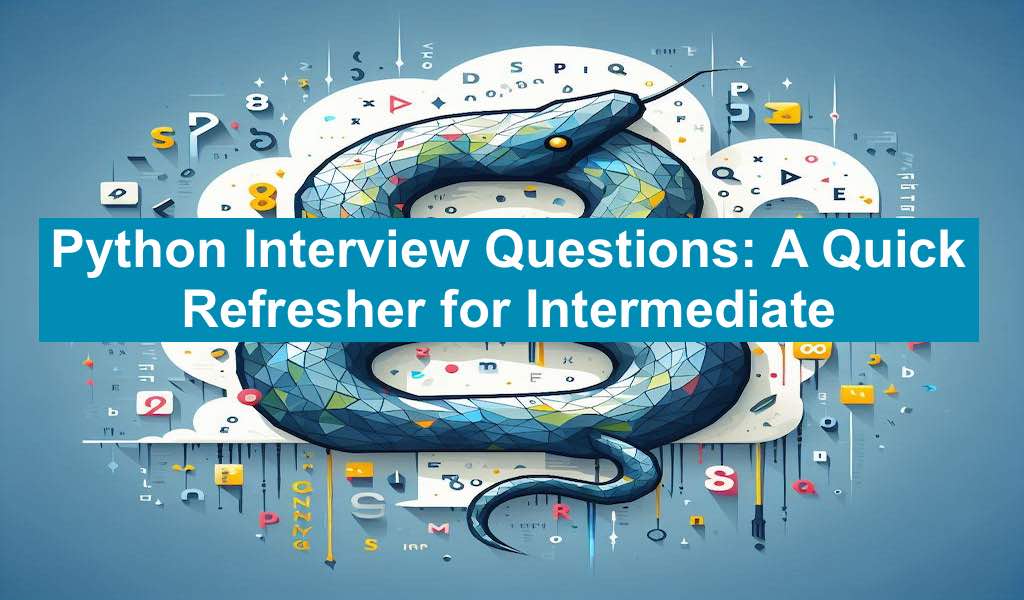
Python interviews assess your knowledge of Python, data structures, libraries, and problem-solving skills. Prepare well with our handpicked questions for intermediate level.
Table of Contents
- 1. What is the difference between xrange and range functions?
- 2. What is dictionary comprehension? Give an Example
- 3. Is tuple comprehension exists? If yes, how, and if not why?
- 4. Differentiate between List and Tuple?
- 5. What is the difference between a shallow copy and a deep copy?
- 6. Which sorting method is employed by Python's sort() and sorted() functions?
- 7. What are Decorators?
- 8. How do you debug a Python program?
- 9. What are Iterators?
- 10. What are Generators?
- 11. Does Python supports multiple Inheritance?
- 12. What is polymorphism?
- 13. Define encapsulation?
- 14. How do you do data abstraction?
- 15. How is memory management done in Python?
- 16. How to delete a file using Python?
- 17. What is slicing?
- 18. What is a namespace in Python?
- Learn more on Python
1. What is the difference between xrange and range functions?
Two Python functions that can be used to iterate a specific number of times in for loops are range() and xrange(). Although xrange has been removed from Python 3, the range function continues to work similarly to xrange in Python 2.
- range() – This function generates a list of numbers and returns it.
- xrange() – This function yields an object generator that can only be used to loop through and display numbers. Because only a specific range is shown when needed, it is referred to as lazy evaluation.
#Syntax start and steps are optional, stop is the only required parameter. Default: start=0, steps=1
range(start, stop, steps)
range() function | xrange() function |
---|---|
In Python-3, the range() method is utilized. | In Python-2, the xrange() method is utilized. |
range() runs faster compare to xrange() | xrange() is slower compared to range() |
An immutable numeric sequence, or list, is returned | A generator object of the xrange type is returned |
The range() is less memory optimized. | The xrange() function has been optimized for additional memory. |
Since the range() function returns a list of numbers, it is compatible with arithmetic operations. | Since it returns a generator object, the xrange() function does not support arithmetic operations. |
range() has the following syntax: range(start, stop, steps) | The xrange() method has the similar syntax: xrange(start, stop, steps) |
2. What is dictionary comprehension? Give an Example
Dictionary Comprehension is a syntax construction to ease the creation of a dictionary based on the existing iterable. So we can say a dictionary comprehension is a method for transforming one dictionary into another dictionary. Items from the original dictionary can be conditionally added to the new dictionary throughout this transformation, and each item can undergo any necessary transformations.

# for example, lists with keys and values
keys = ['a','b','c','d','e']
values = [1,2,3,4,5]
# dict comprehension here
myDict = { k:v for (k,v) in zip(keys, values)}
# We can use below too
# myDict = dict(zip(keys, values))
print (myDict)
3. Is tuple comprehension exists? If yes, how, and if not why?
(i for i in (1, 2, 3))
Python does not support tuple comprehension since the result is a generator rather than a tuple comprehension.
4. Differentiate between List and Tuple?
Let’s analyze the differences between List and Tuple:
List
- Lists are Mutable datatype.
- Lists consume more memory
- The list is better for performing operations, such as insertion and deletion.
- The implication of iterations is Time-consuming
Tuple
- Tuples are Immutable datatype.
- Less memory is used by tuples than by lists.
- It is appropriate to use a Tuple data type to access the elements.
- The implication of iterations is comparatively faster
5. What is the difference between a shallow copy and a deep copy?
A shallow copy builds a new compound object and then adds references to the original objects (as much as possible) in it. Where as a deep copy builds a new compound object and then copies the objects from the original into it in a recursive manner.
Changes made to the new or copied object in the original object are reflected in the Shallow Copy. Changes made to the new or copied object in the original object are not reflected in the deep copy.
Program execution is faster in shallow copies, which store references to any complex object (such as lists within lists, etc.), while deep copies slows down the program execution.
6. Which sorting method is employed by Python’s sort() and sorted() functions?
Python sorts data using the Tim Sort algorithm. The worst case for this stable sorting is O(N log N). It is a hybrid and stable sorting algorithm that works well with a variety of real-world data types. It is derived from merge sort and insertion sort.
7. What are Decorators?
Decorators are a very powerful and useful tool in Python as they allows programmers to modify the behaviour of a function or class. It means decorators allows a user to add a new functionality to an existing object without modifying its structure.
Commonly used decorators like @classmethod, @staticmethod, and @property that are included in Python.
A decorator function is generally an outer function which takes a function (function to be modified) as an input and a wrapper function that the decorator function return with added functionalities to input function.
#Syntax of a decorator function
def my_decorator_func(func):
def wrapper_func():
# Do something before the function.
func()
# Do something after the function.
return wrapper_func
8. How do you debug a Python program?
By using this command we can debug a program:
$ python -m pdb python-script.py
9. What are Iterators?
An object that has the ability to be iterated upon, or travel through every value, is called an iterator.
In Python, one uses an iterator to iterate a collection of elements, such as containers like a list. Iterators are collections of items, which can be dictionaries, lists, or tuples. To iterate across the stored elements, a Python iterator implements __itr__ and the next() function. To iterate over the collections (list, tuple), we often utilize loops.
10. What are Generators?
In Python, a generator is a function that uses the Yield keyword to return an iterator.
The generator is a method that describes how to implement iterators in Python. It is a typical function with the exception of yielding expression in the function. The next() function and __itr__ are not implemented, and additional overheads are also decreased.
A function becomes a generator if it has at least one yield statement. By preserving its states, the yield keyword pauses the current execution and allows it to resume from there as needed.
def generator_function():
yield statement
11. Does Python supports multiple Inheritance?
In contrast to Java, Python does enable multiple inheritances. A class may inherit from more than one parent class thanks to multiple inheritances.
12. What is polymorphism?
Being polymorphic refers to having numerous forms. It is therefore possible for the child class to have a method with the same name, XYZ, but with different parameters and variables if the parent class has a method with the same name. Python is polymorphism-friendly.
13. Define encapsulation?
Encapsulation refers to the binding of data and code together. A prime example of encapsulation is a Python class.
14. How do you do data abstraction?
Data Abstraction conceals the implementation from public view and only provides the necessary information. Abstract classes and interfaces can be used to do that.
15. How is memory management done in Python?
Python manages memory by using its private heap space. The private heap area is essentially where all of the objects and data structures are kept. Because the interpreter looks after this secret area, not even the programmer can access it. Additionally, it features an integrated garbage collector that recycles all of the RAM that isn’t being used and releases it to the heap.
16. How to delete a file using Python?
Using Python, we can remove a file using the following two methods:
- os.remove()
- os.unlink()
17. What is slicing?
A string operation called “slicing” can be used to extract a portion of a list or a portion of a string. This operator allows you to set the step, where to stop the slicing, and where to start. Using list slicing, a new list is created from an old one.
Syntax: list_1[ Start : End : Step ]
18. What is a namespace in Python?
A naming system called a namespace is used to ensure that names are distinct in order to prevent naming disputes. A namespace is a grouping of officially specified symbolic names and the details of the objects they relate to. A namespace can be compared to a dictionary where the objects themselves are the values and the object names are the keys. Local objects and their values are found in the local namespace, whereas global objects and their values are found in the global namespace. Prior to raising an exception, name resolution looks for objects in local namespaces, global and then built-in respectively.
Learn more on Python
- Test Your Knowledge on Python Lists
- A Quick Refresher on Python Interview: A Beginner’s Guide
- Python Interview Questions: A Quick Refresher for Expert
- Python Core Concepts in 10 Mins
- Learn Python on Official Website
- Python List Comprehension: Get Your Code Simplified Today