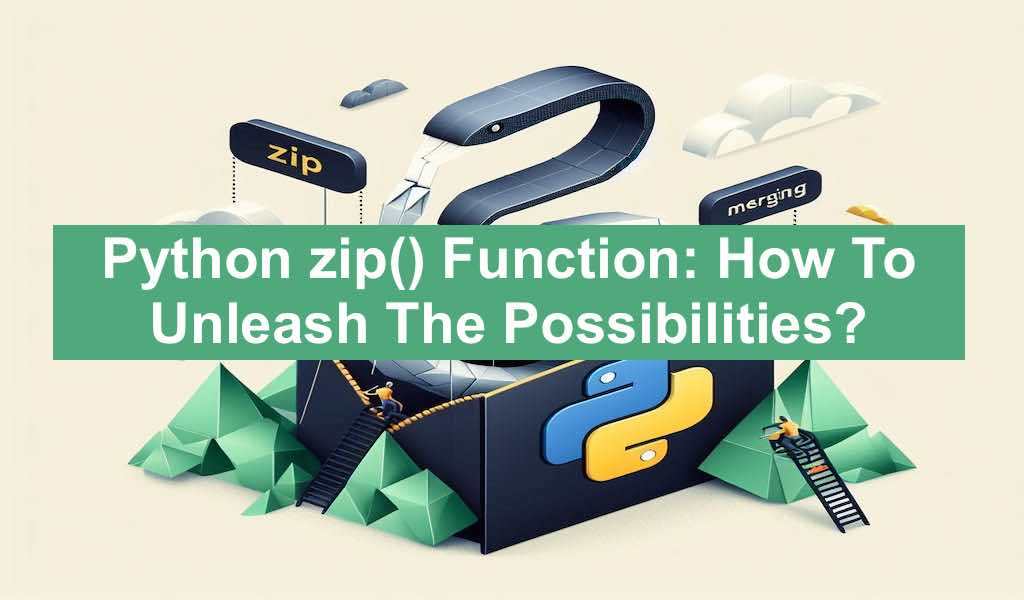
The Python zip() function is a versatile built-in tool that allows you to iterate over multiple iterables simultaneously. It’s a game-changer when it comes to working with related sequences, making it easier to perform operations on corresponding elements across multiple lists, tuples, or other iterable objects.
Table of Content
- Introduction
- Definition
- Examples
- zip() with No Inputs:
- Output of zip() can be Converted to Other iterables:
- Using zip() with Lists:
- Combining zip() with enumerate():
- Creating Dictionary using zip():
- Pairing Tuples with zip():
- Handling Multiple Iterables:
- Handling Lists of Unequal Size:
- Unzip the iterable using zip():
- Perform Parallel Operation:
- Summary
- FAQs
- Q1. What is the zip() function in Python?
- Q2. How does the zip() function handle iterables of different lengths?
- Q3. Can the zip() function be used to unzip a list of tuples?
- Q4. How can you convert the zip() iterator to a list or other data structure?
- Q5. What are some practical applications of the zip() function?
- Q6. Can you use the zip() function with infinite iterators?
- Learn more about related or other topics
Introduction
Are you ready to dive into the world of Python’s zip() function? Brace yourself for a journey through one of Python’s most versatile tools for data manipulation and iteration. Whether you’re a novice or a seasoned Pythonista, understanding zip() can elevate your coding prowess to new heights.
Definition
At its core, the zip() function takes two or more iterables as arguments and returns an iterator of tuples. Each tuple contains the corresponding elements from the provided iterables. If the iterables have different lengths, the zip() function will stop iterating when the shortest iterable is exhausted.
Syntax
#can have zero or more input iterables
zip(iterable1, iterable2, iterable3,...)
Examples
Let’s dive into the zip()
function in Python. This versatile function allows you to combine multiple iterables (such as lists, tuples, or dictionaries) into a single iterable. It pairs elements from corresponding positions, creating tuples. Here are some examples to illustrate its usage:
zip() with No Inputs:
# No inputs to zip function
print(zip())
#Output: <zip object at 0x7f91a2b9f3c0>
#Converting zipped object to list, as no input passed to zip a empty list created
print(list(zip()))
#Output: []
Output of zip() can be Converted to Other iterables:
Zip() function return an iterable, we can use iterable type function to convert it to that type.
emp_names_tuple = ("Ajay", "Kunal", "Ashish", "Rahul")
emp_doj_tuple = ('25-10-2023', '12-06-2018', '01-02-202', '21-09-2022')
zipped = zip(emp_names_tuple, emp_doj_tuple)
print(zipped)
#Output : <zip object at 0x7f91a2ba2240>
#Converting to list
list_result = list(zip(emp_names_tuple, emp_doj_tuple))
print(list_result)
#Output : [('Ajay', '25-10-2023'), ('Kunal', '12-06-2018'), ('Ashish', '01-02-202'), ('Rahul', '21-09-2022')]
#Converting to tuple
tuple_result = tuple(zip(emp_names_tuple, emp_doj_tuple))
print(tuple_result)
#Output : (('Ajay', '25-10-2023'), ('Kunal', '12-06-2018'), ('Ashish', '01-02-202'), ('Rahul', '21-09-2022'))
#Converting to set
set_result = set(zip(emp_names_tuple, emp_doj_tuple))
print(set_result)
#Output : {('Kunal', '12-06-2018'), ('Ashish', '01-02-202'), ('Rahul', '21-09-2022'), ('Ajay', '25-10-2023')}
Using zip()
with Lists:
Suppose we have two lists: emp_names and emp_id
. We can use zip()
to pair corresponding elements from these lists:
emp_names = ["Ajay", "Kunal", "Ashish", "Rahul"]
emp_id = [100, 101, 103, 102]
zipped_lists = zip(emp_names, emp_id)
print(list(zipped_lists))
#Output
#[('Ajay', 100), ('Kunal', 101), ('Ashish', 103), ('Rahul', 102)]
Combining zip()
with enumerate()
:
When you want to process multiple lists or tuples in parallel and need access to their indices, use zip()
along with enumerate()
:
emp_names = ["Ajay", "Kunal", "Ashish", "Rahul"]
emp_ages = [35, 30, 28, 49]
for indx, (emp_names, emp_ages) in enumerate(zip(emp_names, emp_ages)):
print(indx, emp_names, emp_ages)
# Output:
#0 Ajay 35
#1 Kunal 30
#2 Ashish 28
#3 Rahul 49
Creating Dictionary using zip()
:
We can create dictionary with 2 different iterables using zip()
functions based on their positions:
emp_names = ["Ajay", "Kunal", "Ashish", "Rahul"]
emp_id = [100, 101, 103, 102]
create_new_dict = {emp_name: emp_id for emp_name, emp_id in zip(emp_names, emp_id)}
print(create_new_dict)
# Output:
# {'Ajay': 100, 'Kunal': 101, 'Ashish': 103, 'Rahul': 102}
One powerful use case of zip() is creating dictionaries from two lists. Instead of using a loop and manually constructing key-value pairs, you can leverage zip() to achieve the same result in a more concise and readable way:
dict_keys = ['name', 'age', 'city']
dict_values = ['Ajay', 35, 'Mumbai']
person = dict(zip(dict_keys, dict_values))
print(person)
#Output
# {'name': 'Ajay', 'age': 35, 'city': 'Mumbai'}
Pairing Tuples with zip()
:
When used with tuples, zip()
pairs elements based on their positions:
emp_names_tuple = ("Ajay", "Kunal", "Ashish", "Rahul")
emp_doj_tuple = ('25-10-2023', '12-06-2018', '01-02-202', '21-09-2022')
tuple_result = tuple(zip(emp_names_tuple, emp_doj_tuple))
print(tuple_result)
#Output : (('Ajay', '25-10-2023'), ('Kunal', '12-06-2018'), ('Ashish', '01-02-202'), ('Rahul', '21-09-2022'))
Handling Multiple Iterables:
zip()
can combine more than two iterables. It returns an iterable of tuples, where each tuple contains elements from corresponding positions:
iter1 = [10, 20, 30]
iter2 = ['aA', 'bB', 'cC']
iter3 = ['xX', 'yY', 'zZ']
zipped = zip(iter1, iter2, iter3)
result = list(zipped)
print(result)
# Output:
#[(10, 'aA', 'xX'), (20, 'bB', 'yY'), (30, 'cC', 'zZ')]
Handling Lists of Unequal Size:
If the input lists have different lengths, zip()
will iterate over the smallest list:
input1 = [10, 20, 30, 40, 50] #length 5
input2 = ['p', 'q', 'r', 't'] #length 4
zipped_list = zip(input1, input2) #zipped upto 4 elements
print(list(zipped_list))
# Output:
[(10, 'p'), (20, 'q'), (30, 'r'), (40, 't')] #length 4
The resulting combination will only be as long as the smallest list.
If you want to get the result should have items from the longest iterable
then you can utilise the itertools
module. In that case the elements for the shortest iterable
will be all None
.
import itertools as it
input1 = [10, 20, 30, 40, 50] #length 5
input2 = ['p', 'q', 'r', 't'] #length 4
zipped_longest_list = it.zip_longest(input1, input2)
print(list(zipped_longest_list))
#Output
[(10, 'p'), (20, 'q'), (30, 'r'), (40, 't'), (50, None)] #length 5, with None for smallest iterable
Unzip the iterable using zip():
The zip() function can also be used with the *
operator to unzip iterables. This allows you to transpose or reverse the zipping process, separating the elements of zipped tuples into individual iterables:
zipped = [('x', 100), ('y', 200), ('z', 300)]
letters, numbers = zip(*zipped)
print(list(letters)) # ['x', 'y', 'z']
print(list(numbers)) # [100, 200, 300]
Perform Parallel Operation:
Another handy application of zip() is parallel operations on multiple iterables. For instance, you can add corresponding elements from two lists without using an explicit loop:
list1 = [10, 20, 30]
list2 = [40, 50, 60]
addition_result = [x + y for x, y in zip(list1, list2)]
print(addition_result)
#Output
# [50, 70, 90]
Summary
The zip() function is incredibly versatile and can be used in various scenarios, such as data preprocessing, data manipulation, and parallel processing. Its ability to iterate over multiple iterables simultaneously makes it a powerful tool in Python’s arsenal, enabling more concise and readable code while enhancing productivity. Remember that zip()
returns an iterator, so you can convert it to a list or use it directly in loops or other operations.
FAQs
Q1. What is the zip() function in Python?
The zip() function is a built-in Python function that takes two or more iterables (such as lists, tuples, or strings) and returns an iterator of tuples. Each tuple contains the elements from each iterable at the corresponding index.
Q2. How does the zip() function handle iterables of different lengths?
The zip() function stops iterating when the shortest iterable is exhausted. If you want to continue zipping with a fill value for the remaining elements, you can use the zip_longest()
function from the itertools
module.
Q3. Can the zip() function be used to unzip a list of tuples?
Yes, you can use the *
operator to unpack the tuples in a list and pass them as separate arguments to the zip() function. This effectively “unzips” the list of tuples into separate lists or iterables.
Q4. How can you convert the zip() iterator to a list or other data structure?
The zip() function returns an iterator, which is a lazy generator. To convert it to a list or other data structure, you can use type constructors like list()
, tuple()
, or dict()
. For example, list(zip(list1, list2))
will create a list of tuples.
Q5. What are some practical applications of the zip() function?
The zip() function is commonly used to iterate over multiple lists or iterables simultaneously, such as merging data from different sources, transposing matrices, or creating dictionaries from two lists (keys and values). It can also be used for data compression and decompression tasks.
Q6. Can you use the zip() function with infinite iterators?
No, the zip() function will not work with infinite iterators because it will try to iterate over them indefinitely. If you use zip() with an infinite iterator, it will cause an infinite loop and potentially hang your program. For infinite iterators, you should use other tools like the itertools
module or generator expressions.
Learn more about related or other topics
- Python List Comprehension: Get Your Code Simplified Today
- Python Interview Questions: A Quick Refresher for Intermediate
- Test Your Knowledge on Python Lists
- Python Interview Questions: A Quick Refresher for Expert
- zip() function by Python.org
- Snowflake Time Travel: How to Make It Work for You?
- NoSQL Vs SQL Databases: An Ultimate Guide To Choose
- AWS Redshift Vs Snowflake: How To Choose?
- SQL Most Common Tricky Questions