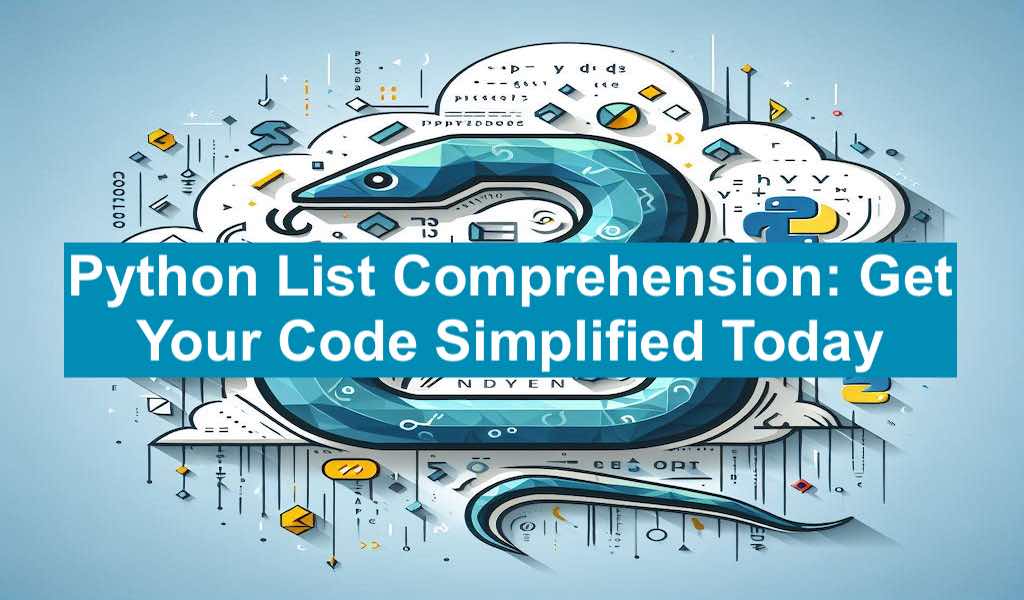
List comprehension is a powerful feature of Python that lets you create new lists from existing ones in a concise and elegant way. List comprehension is one of the most powerful tools in Python. Lets dive into it in details
Table of Content
Introduction
List comprehension in Python provides a simple and compact syntax for converting any other iterable, like a string or another list, into a list. It’s a fairly succinct method of creating a new list by applying a function to each entry in the current list. Comparing list comprehension to the for loop method of list processing, the latter is more slower.
For example, consider the below list of cloud service providers, if you want to create a new list for all cloud provider name contains ‘a’ then you need to write a for loop with the if condition.
cloud_providers = ["AWS", "Google", "Microsoft Azure", "IBM", "AliBaba","Oracle"]
newlist = []
for x in cloud_providers:
if "a" in x:
newlist.append(x)
print(newlist)
Output:
['AliBaba', 'Oracle']
But using list comprehension you can do it all in single line of code.
cloud_providers = ["AWS", "Google", "Microsoft Azure", "IBM", "AliBaba","Oracle"]
newlist = [x for x in cloud_providers if "a" in x]
print(newlist)
Output:
['AliBaba', 'Oracle']
Syntax of List Comprehension

Square brackets, [], are used to begin and conclude list comprehensions. Next, you specify the expression or action you want to apply to each value in the current iterable. These computations’ outcomes are added to a new list. An optional if clause and a for clause come after the expression.
- expression: The expression is the action we take on the iteration’s current item. It is the expression’s output that is utilized to generate a new list. It can also have conditions in order to alter the outcome rather than only act as a filter.
- for loop: can have one or more for loops to iterate over any iterable
- iterable: any iterable objects for example list, string, tuple, set, even can use range(n,m) can be used
- condition: One or more optional conditions may be applied. The condition works as a filter, allowing only elements that evaluate to True to be accepted.
List Comprehension using multiple loop (nested loops)
As mentioned earlier, we can use nested loops in a list comprehension. For example, if we need all combinations of items from two lists in the form of a tuple then we can use list comprehension as mentioned in below code. Here we use two for loop to iterate over both the lists (number_list1 & number_list2), for each number in number_list1 second loop is running on number_list2 to get the second number in the tuple.
number_list1 = [10, 20, 30]
number_list2 = [70, 80, 90]
list_contains_tuples =[(x,y) for x in number_list1 for y in number_list2]
print(list_contains_tuples)
Output:
[(10, 70), (10, 80), (10, 90), (20, 70), (20, 80), (20, 90), (30, 70), (30, 80), (30, 90)]
Using multiple conditions
Similar to using multiple loops in list comprehension, we can use multiple conditions to filter the result of output expression. For example, in below code we are looking for all numbers lesser than 100 which are divisible by both 3 & 5 i.e. all numbers less than 100 are divisible by 15. here we are using two conditions, checking each number whether it is divisible by 3 and 5 simultaneously.
number_list = [x for x in range(100) if x%3==0 if x%5==0]
print(number_list)
Output:
[0, 15, 30, 45, 60, 75, 90]
List Comprehension with if-else in output expression
Even in the list comprehension output expression, if-else can be used. The sole distinction in use if-else in the output expression is that all records are obtained based on the number of items in the iterable; no filtering is applied. However, when we employ if-else in a condition clause, filtering takes place in accordance with the clause, and we can receive fewer records than the iterable’s total number of items.
Let’s consider the example if given number is divisible by 12 or not.
Note: Any number is divisible by 12 if that number is divisible by both 3 and 4. The reason of it is because least common multiple (LCM) of 3 and 4 is 12.
number_list = [ str(x) + ' is divisble by 12' if x%3==0 and x%4==0 else str(x) + ' is not divisible by 12' for x in range(10,37) ]
print(number_list)
Output:
['10 is not divisible by 12', '11 is not divisible by 12', '12 is divisble by 12', '13 is not divisible by 12', '14 is not divisible by 12', '15 is not divisible by 12', '16 is not divisible by 12', '17 is not divisible by 12', '18 is not divisible by 12', '19 is not divisible by 12', '20 is not divisible by 12', '21 is not divisible by 12', '22 is not divisible by 12', '23 is not divisible by 12', '24 is divisble by 12', '25 is not divisible by 12', '26 is not divisible by 12', '27 is not divisible by 12', '28 is not divisible by 12', '29 is not divisible by 12', '30 is not divisible by 12', '31 is not divisible by 12', '32 is not divisible by 12', '33 is not divisible by 12', '34 is not divisible by 12', '35 is not divisible by 12', '36 is divisble by 12']
Flattening the list of lists using List Comprehension
Flattening a list that contains another lists is one of the use case for the List Comprehension with multiple loop method.
list_contains_lists =[['a', 'b', 'c'], ['d','e','f'], ['g','h','i','j']]
flattened_single_list=[chr for inner_list in list_contains_lists for chr in inner_list]
print(flattened_single_list)
Output:
['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j']
List Comprehension Advantages and Disadvantages
Advantages
- Lesser code to write making code look clean and professional
- Faster than the for loop
Disadvantages
- Readability issue, as the code is shorter it may be difficult to read and understand, specially with multiple loops or conditions
- Debugging may be difficult for some complex list comprehensions
Summary
List comprehensions are a great way to create new lists according to your needs. They also have the added advantage of making your code appear tidy and professional. They are far faster than loop.
The only concern with list comprehension is that it is less readable also one has to put extra efforts to understand and debug it if any issue occur. Although it can make your code shorter, it also makes it harder to read, particularly as the expressions and conditions are complicated. Since list comprehension just involves one line of code, debugging it can be challenging for certain programmers.
You also have access to numerous list comprehensions in cases where you’re working with nested lists. At first, the idea of employing comprehensions might seem a little complicated, but once you master it, you won’t turn back!
Learn more about Python
- Python Interview Questions: A Quick Refresher for Intermediate
- Test Your Knowledge on Python Lists
- Python Interview Questions: A Quick Refresher for Expert
- Python List Comprehensions